東京大学 工学系研究科 電気系工学専攻 2020年度 問題4 情報工学II
Author
Description
I.
Answer the following questions on sequential circuits. We consider a circuit that has four states \(S=\{s0, s1, s2, s3\}\). The circuit changes its state and output \(Y\) according to the value of input \(X\). Table \(1\) shows the state transition table of the circuit.
(1) Draw the state transition diagram that is equivalent to the state transition table in Table \(1\).
(2) Show the shortest input sequence that outputs \(Y=1\) when the initial state is \(s2\).
Next, assume that we represent the state S with two bits as \(s0=00\), \(s1=01\), \(s2=10\), \(s3=11\). We consider designing the circuit with two JK-Flip Flop (JK-FF) \(F_A\) and \(F_B\).We represent the higher bit of the states with \(Q_A\), and the lower bit with \(Q_B\) such as \(s=Q_AQ_B\). \(F_A\) and \(F_B\) hold the states \(Q_A\) and \(Q_B\) in the circuits, respectively. A JK-FF has one bit state Q, and the state transits as shown in Fig. \(1\) according to inputs J and K.
(3) Copy Table \(1\) to your answer sheet and complete the table by filling the blanks. Here, (\(J_A, K_A\)) and (\(J_B, K_B\)) are the inputs to \(F_A\) and \(F_B\), respectively. Use \(d\) for "don't care" conditions.
(4) Simplify the input functions of \(J_A, K_A, J_B, K_B\) and output function of \(Y\) by using Karnaugh maps.
(5) Draw the circuit using the symbols shown in Fig. \(2\).
Table 1
Current state \(S(t)\) | Input \(X\) | Next state \(S(t+1)\) | Output \(Y\) | \(Q_A(t)\) | \(Q_B(t)\) | \(Q_A(t + 1)\) | \(Q_B(t + 1)\) | J_A | K_A | J_B | K_B |
---|---|---|---|---|---|---|---|---|---|---|---|
\(s_0\) | 0 | \(s_1\) | 0 | ||||||||
\(s_0\) | 1 | \(s_2\) | 0 | ||||||||
\(s_1\) | 0 | \(s_3\) | 0 | ||||||||
\(s_1\) | 1 | \(s_2\) | 0 | ||||||||
\(s_2\) | 0 | \(s_0\) | 0 | ||||||||
\(s_2\) | 1 | \(s_2\) | 0 | ||||||||
\(s_3\) | 0 | \(s_0\) | 0 | ||||||||
\(s_3\) | 1 | \(s_2\) | 1 |
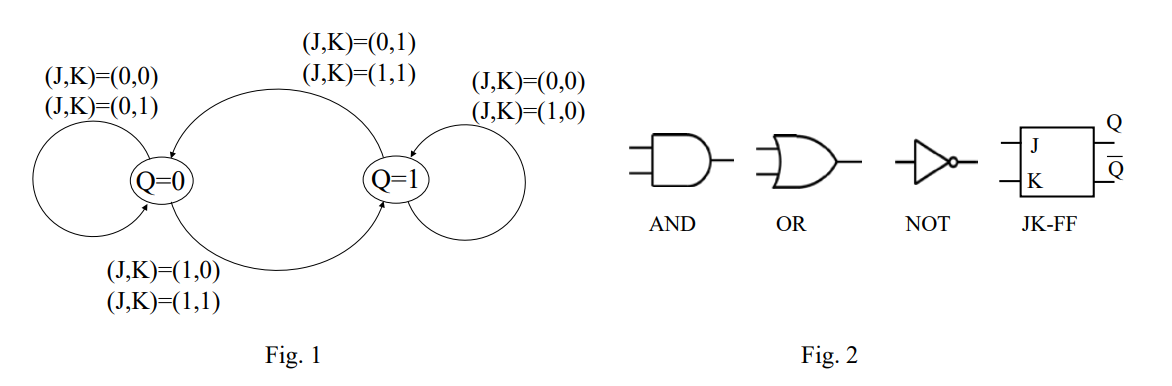
II.
Answer the following questions on heapsort. Assume that max heap is an array representation of a binary tree where the value in a parent node is greater than the values in its two children nodes. The structure of the array is as follows: assuming that the index \(i\)is an integer greater than or equal to \(1\), the value in the left child node of node \(i\) is stored in the \((i \times 2)\)-th element of the array, and the value in the right child node of node \(i\) is stored in the \((i \times 2 + 1)\)-th element of the array.
The function make_heap
in Program \(1\) is a function that generates the max heap from an array
with n
items.The first element of the array is stored in array[1]
.The function swap(array,i,j)
in Program \(1\) is a function that switches the values of array[i]
and array[j]
.Here,the values in the array are integers,and there is no duplication among them.
(1) Assume that the number of items is \(7 (n=7)\), and the input array
stores the values \(\{1, 7, 8, 9, 6, 4, 5\}\) in this order. Find the max heap that is generated by make_heap
in Program \(1\).
(2) Draw the process of generating the max heap in Question (1) with binary trees. The arrays follow the same structure as the max heap even during the process of making max heap. You do not need to draw the trees that remain unmodified in the process.
(3) Give the order of the time complexity of make_heap
according to the number of comparisons of the values.
Assume that we sort a max heap by iteratively taking the maximum value and rebuilding a max heap with the remaining items.
(4) Describe a function by using the \(C\) programming language in as few lines as possible to sort a max heap array
with n
items in descending order. Use the function make_heap
in Program \(1\) for rebuilding the max heap in your function.
(5) Describe an overview of an algorithm to sort an array using max heap, which is more computationally efficient than that obtained in Question (4). The sort order can be either ascending order or descending order.
Kai
I.
(1)
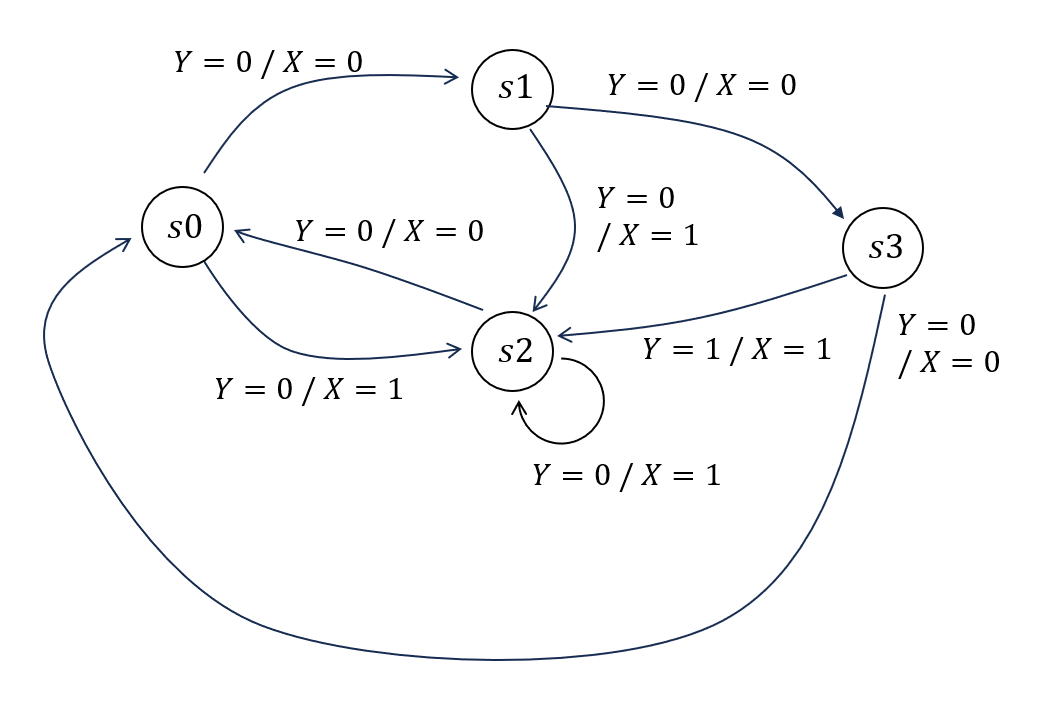
(2)
\(x = 0,0,0,1\) の順
(3)
Current state \(S(t)\) | Input \(X\) | Next state \(S(t+1)\) | Output \(Y\) | \(Q_A(t)\) | \(Q_B(t)\) | \(Q_A(t + 1)\) | \(Q_B(t + 1)\) | J_A | K_A | J_B | K_B |
---|---|---|---|---|---|---|---|---|---|---|---|
\(s_0\) | 0 | \(s_1\) | 0 | 0 | 0 | 0 | 1 | 0 | \(d\) | 1 | \(d\) |
\(s_0\) | 1 | \(s_2\) | 0 | 0 | 0 | 1 | 0 | 1 | \(d\) | 0 | \(d\) |
\(s_1\) | 0 | \(s_3\) | 0 | 0 | 1 | 1 | 1 | 1 | \(d\) | \(d\) | 0 |
\(s_1\) | 1 | \(s_2\) | 0 | 0 | 1 | 1 | 0 | 1 | \(d\) | \(d\) | 1 |
\(s_2\) | 0 | \(s_0\) | 0 | 1 | 0 | 0 | 0 | \(d\) | 1 | 0 | \(d\) |
\(s_2\) | 1 | \(s_2\) | 0 | 1 | 0 | 1 | 0 | \(d\) | 0 | 0 | \(d\) |
\(s_3\) | 0 | \(s_0\) | 0 | 1 | 1 | 0 | 0 | \(d\) | 1 | \(d\) | 1 |
\(s_3\) | 1 | \(s_2\) | 1 | 1 | 1 | 1 | 0 | \(d\) | 0 | \(d\) | 1 |
(4)
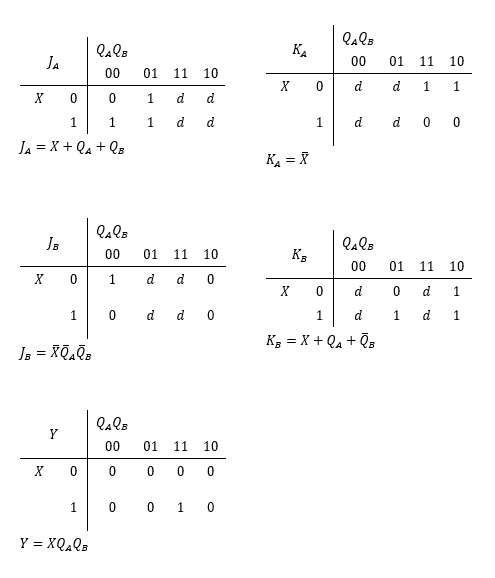
(5)
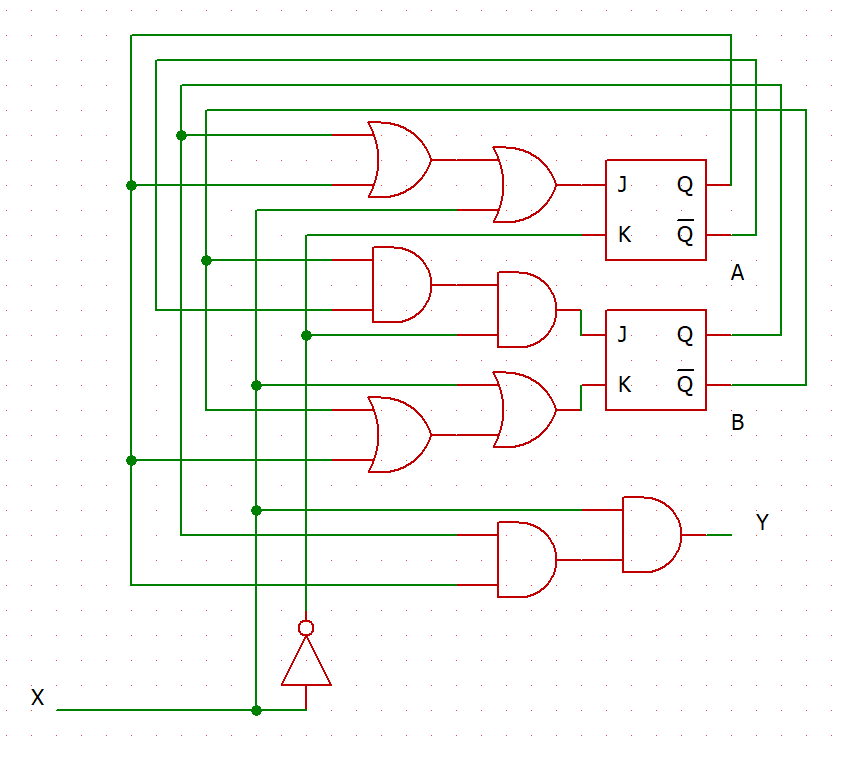
II.
(1)
\(\{9,7,8,1,6,4,5\}\)
問題のコードはC言語と違って配列のインデックスが1スタートなので注意。
(2)
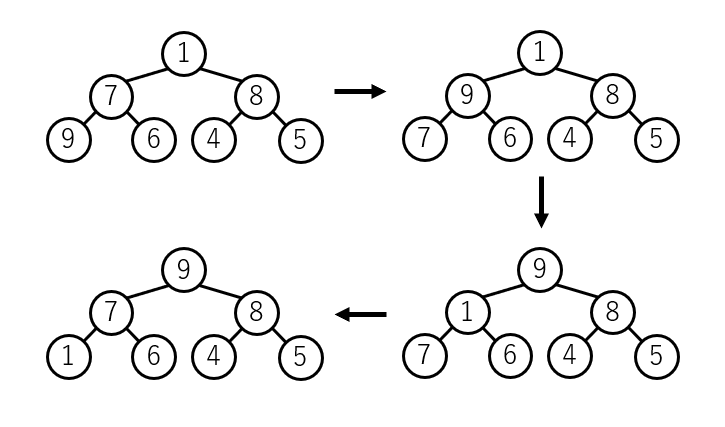
(3)
要素の数を \(n\) として、\(O(n \log n)\)
(4)
本解:
本解を改行したもの:
参考解:
ヒープソートの話かなと思ったので最初参考解を思いついたが、as few lines as possible
という問題文の指示が気になったので本解の方を思いついた。もっとも、どんなプログラムであれ改行無しで書けば \(1\) 行になるのだが。
どちらもmake_heap
を実行するとarray
の先頭が最大値になることを利用している。以下は本解の解説。
make_heap
の引数は先頭アドレスarray + i
、サイズn - i
の配列を渡すという意味である。本来先頭アドレスarray
、サイズn
の配列から部分配列を切り出してmake_heap
に渡す操作を行っている。ちなみに配列はメモリ上で、(インデックスが \(1\) スタートのこのプログラムにのっとって言うと、)アドレスarray
にarray[1]
、array + 1
にarray[2]
、...、array + n - 1
にarray[n]
という風に配置されている。
for
文の()の中の書き方のコツはイテレータ変数(解答でいうとi
)の初期値と最終値だけ見ることである。今回は初期値がi = 0
で、条件式i < n - 1
がi = n - 2
の時まではtrue
になることから最終値がi = n - 2
になることがわかる。make_heap
に渡した引数の方も初期値と最終値だけ考えればよく、初期値が(array, n)
、最終値が(array + n - 2, 2)
であることがわかる。別にi = n - 1
まで回してもいいのだが、その時はサイズ \(1\) の配列をmake_heap
することになり何も起こらないので条件式をi < n - 1
に設定している。ちなみにi <= n - 2
としてもよい。こちらの方が直感的かもしれない.
(5)
仰々しく書いてしまったが要はヒープソートをやっている。コードで書くと次のよう。
計算量オーダーは (4) が \(O(n^2 \log n)\)、(5) が \(O(n \log n)\) なので (5) の方が効率的になっている。