東北大学 工学研究科 電気・情報系 2015年8月実施 問題4 情報基礎2
Author
祭音Myyura
Description
日本語版
下記の条件を満たす
- (条件) 各節点
に対し、 の要素を とするとき、 の左部分木内の要素はすべて より小さ く、 の右部分木内の要素はすべて さより大きい。
各節点の要素とは重複しない整数であるとし、以下の問に答えよ。
(1) Fig. 4 は空の
(2) Fig. 4 の
(3) ある
(4) ある
English Version
A binary tree that satisfies the following condition is called a binary search tree.
- (Condition) For each node
, let be the element of , each element stored in the left sub-tree of is smaller than , and each element stored in the right sub-tree of is greater than .
Assume that the element
(1) Fig. 4 shows a binary search tree
(2) Delete the node which stores the element
(3) Give an algorithm to delete a node
(4) Describe an efficient algorithm to enumerate all elements of a binary search tree in ascending order.
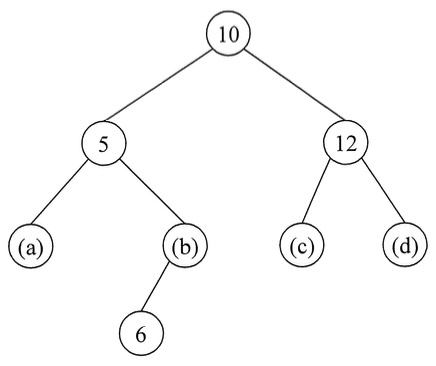
Kai
(1)
2 8 11 15
(2)
8
/ \
5 12
/ \ / \
2 6 11 15
or
11
/ \
5 12
/ \ \
2 8 15
/
6
(3)
func minValue(root)
minv = root->key
while root->left do
minv = root->left->key
root = root->left
return minv
func deleteNode(root, key)
if root == NULL then
return root
if key < root->key then
root->left = deleteNode(root->left, key)
else if key > root->key then
root->right = deleteNode(root->right, key)
else
if root->left == NULL then
return root->right
elif root->right == NULL then
return root->left
root->key = minValue(root->right)
root->right = deleteNode(root->right, root->key)
return root
(4)
Hint: The inorder traversal of a BST gives the values of the nodes in sorted order.
func inorder(root):
if root != NULL then
inorder(root->left)
print(root->key)
inorder(root->right)